Sleeping and Waking Up
Transitions by a thread that is sleeping and waking up are illustrated in Figure 22.8.
A call to the static method sleep() in the Thread class will cause the currently running thread to temporarily pause its execution and transit to the TIMED_WAITING state. The getState() method on this thread will return the value Thread.State.TIMED_WAITING.
The thread is guaranteed to sleep for at least the time specified in its argument, before transitioning to the READY substate where it takes its turn to run again. It might sleep a little longer than the specified time, but it will not sleep indefinitely.
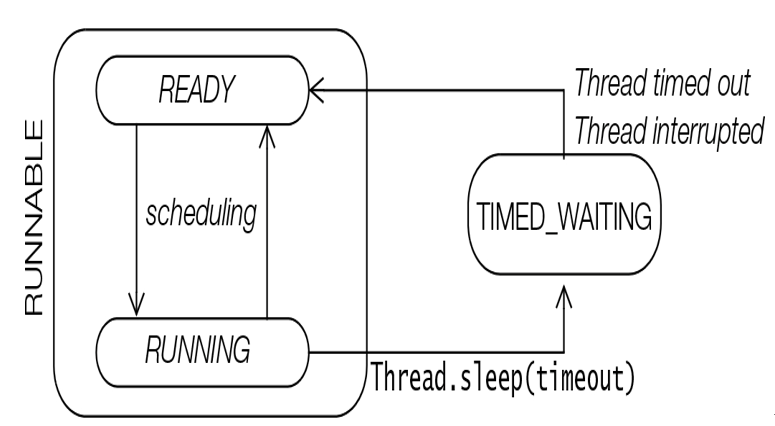
Figure 22.8 Sleeping and Waking Up
If a thread is interrupted while sleeping, it will throw a checked Interrupted-Exception when it awakes and gets to execute. The idiom is to call the sleep() method in a try-catch block that handles the InterruptedException. The sleep() method does not release any locks that the thread might have.
There are two overloaded versions of the sleep() method in the Thread class, allowing time to be specified in milliseconds, and additionally in nanoseconds.
Usage of the sleep() method is illustrated in Examples 22.1, 22.2, and 22.4.
Thread Coordination
Before proceeding further with thread coordination using the wait() and notify()/ notifyAll() methods, it must be emphasized that these methods provide low-level thread coordination, which is error-prone and difficult to get right, and should be avoided in favor of the high-level concurrency support provided by the java.util.concurrent package (Chapter 23, p. 1419).
Waiting and notifying provide a means of coordination between threads that synchronize on the same object—also called data access synchronization. The threads execute wait() and notify() (or notifyAll()) methods on the shared object for this purpose. These final methods are defined in the Object class, and therefore are inherited by all objects. These methods can only be executed on an object whose lock the thread holds (i.e., in synchronized code); otherwise, the call will result in an IllegalMonitorStateException.
final void wait(long timeout) throws InterruptedException
final void wait(long timeout, int nanos) throws InterruptedException
final void wait() throws InterruptedException
A thread invokes the wait() method on the object whose lock it holds. The thread is added to the wait set of the current object.
final void notify()
final void notifyAll()
A thread invokes a notification method on the current object whose lock it holds to notify thread(s) that are in the wait set of the object.